Format Preserving Encryption with Go
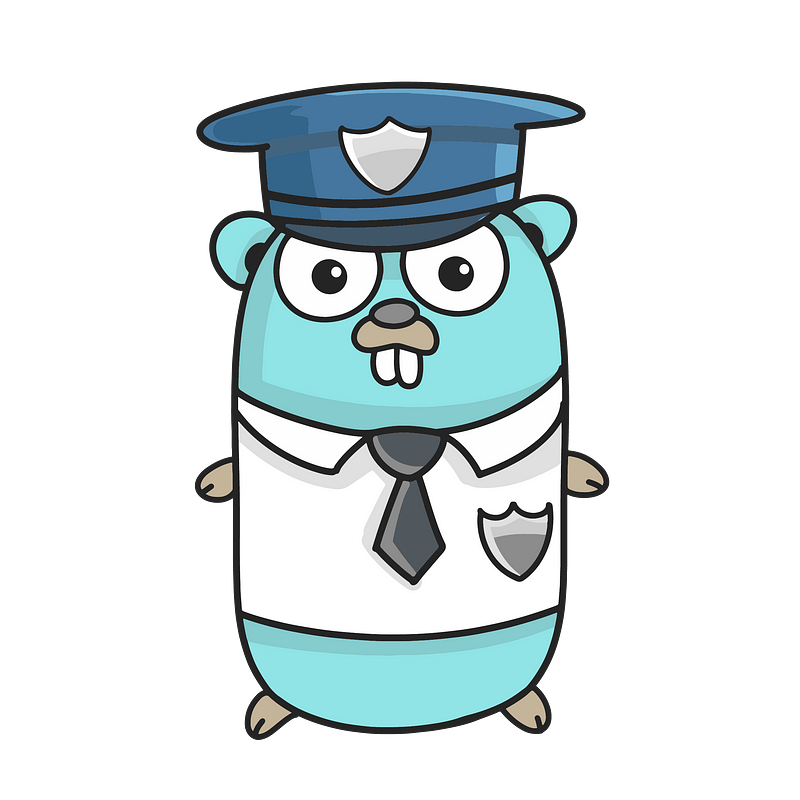
Format Preserving Encryption with Go
Within tokenization, we can apply format-preserving encryption (FPE) methods, which will convert our data into a format which still looks valid, but which cannot be mapped to the original value. For example, we could hide Bob’s credit card detail into another valid credit card number, and which would not reveal his real number. A tokenization server could then convert the real credit card number into a format which still looked valid. For this, we have a key which takes the data and then converts it into a form which the same length as the original.
The method we use is based on a Feistel structure, and where we have a number of rounds, and then apply the key through a Feistel function for each round:

We thus split the data into blocks (typically 64-bits), and then split into two parts. We then take these splits into the left part and the right part, and feed through each round, and then swap them over. The ⊕ symbol is an exclusive-OR operator.
An example of the Friestel cipher is defined here.
Format-preserving, Feistel-based encryption
So, we have a problem here. In most encryption methods we deal with block sizes, such as 64 bit for DES and 128 bits for AES. The output will then be a multiple of 64 bits or 128 bits, as we cipher one block at a time. In FPE we want to have something which will match to the length of the input data. The solution is Format-preserving, Feistel-based encryption (FFX) and which produces an output which matches the length of the input.
NIST has thus defined a standard known as SP 800–38G, and which defines two FF schemes: FF1 and FF3. While these work on 128-bit block sizes, they can also work on blocks which have fewer bits than this. For this we have a key (K) and which creates a permutation of the bits to create an invertible version of the output.
For FF1 we have 10 rounds and for FF3 we have eight rounds. First, we split an input value of n characters into a number of characters (u and v — and where n = u + v):

For the encrypting process we use a modular addition (EX-OR) and for decryption, we use a modular subtraction. For each round, we split into a and b. For the F function in each round, we generate an HMAC output (using SHA-1) from the key (K), the bᵢ, and the counter value (i).
An outline of the code is:
A sample run is:
Message: Hello
Number of chars: 52
Passphrase: qwerty
Salt: 52fdfc072182654f
Cipher: zrhcq
Decipher: Hello
In 2017, Betül Durak (Rutgers University) and Serge Vaudenay (Ecole Polytechnique Fédérale de Lausanne) defined a cryptanalytic attack on the FF3 technique for format-preserving encryption (FPE) [here]. NIST now do not recommend it for general-purpose FPE.

Here is FFX1 in action:
What a 20th Century world we live in, and where we still store sensitive identifiers for citizens, and which map to…asecuritysite.com
and with Node.js: