Blocks To Go …
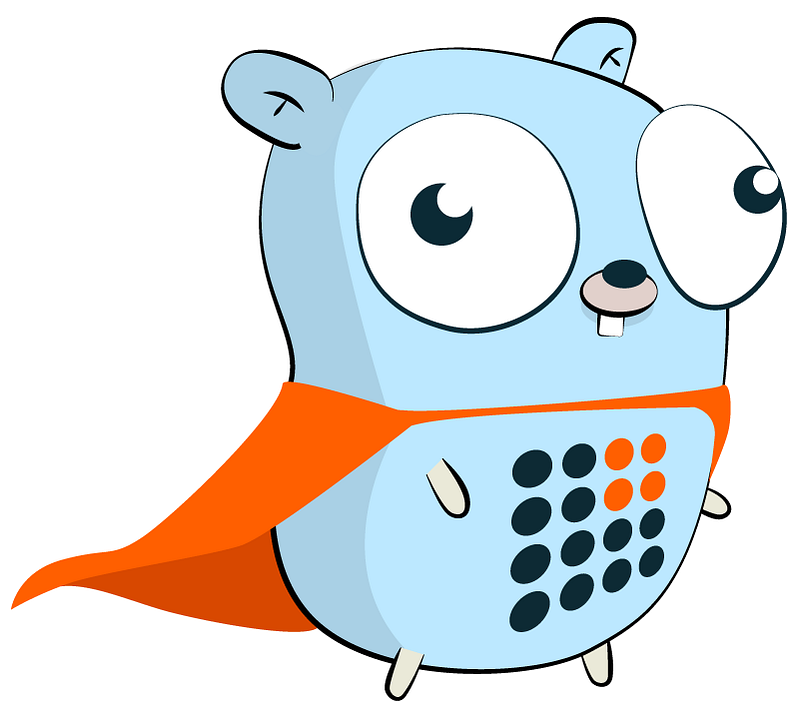
Blocks To Go …
With block encryption we take blocks of data and encrypt each block. DES, for example, uses a 64-bit block size (8 bytes) and AES uses a 128-bit block size (16 bytes). Often the data will not fill all of the blocks so we need to pack bytes into the data. We then encrypt, and then decrypt. After this we then unpad the padding bytes. Three common methods are:
- PKCS7. This pads with the value which equals the number of padding bytes.
- ANSI X.923. This pads with zero until the last byte, and which is the number of padding bytes).
- ISO 10126. This pads with random bytes until the last byte, and which defines the number of padding bytes.
So let’s use Golang to create a demo [here]:
package main
import (
"strconv"
"github.com/enceve/crypto/pad"
"fmt"
"os"
)
func main() {
msg:="Hello"
argCount := len(os.Args[1:])
blocksize := 16
if (argCount>0) {msg = string(os.Args[1])}
if (argCount>1) {blocksize,_ = strconv.Atoi(os.Args[2])}
m := []byte(msg)
pkcs7 := pad.NewPKCS7(blocksize)
pad1 := pkcs7.Pad(m)
fmt.Printf("PKCS7 Pad:\t%x",pad1)
res,_:=pkcs7.Unpad(pad1)
fmt.Printf("\n Unpad:\t%s",res)
x923 := pad.NewX923(blocksize)
pad2 := x923.Pad(m)
fmt.Printf("\n\nX923 Pad:\t%x",pad2)
res,_=x923.Unpad(pad2)
fmt.Printf("\n Unpad:\t%s",res)
ISO10126 := pad.NewISO10126(blocksize,nil)
pad3 := ISO10126.Pad(m)
fmt.Printf("\n\nXISO1012 Pad:\t%x",pad3)
res,_=ISO10126.Unpad(pad3)
fmt.Printf("\n Unpad:\t%s",res)
}
A sample run is [here]:
Block size: 8 bytes 64 bits
PKCS7 Pad: 71776572747931323307070707070707
Unpad: qwerty123
X923 Pad: 71776572747931323300000000000007
Unpad: qwerty123
XISO1012 Pad: 7177657274793132330a30e0bcdd0e07
Unpad: qwerty123
Conclusions
Here’s a quick demo of padding: