The Most Horrible and Amazing Lanaguge: JavaScript!
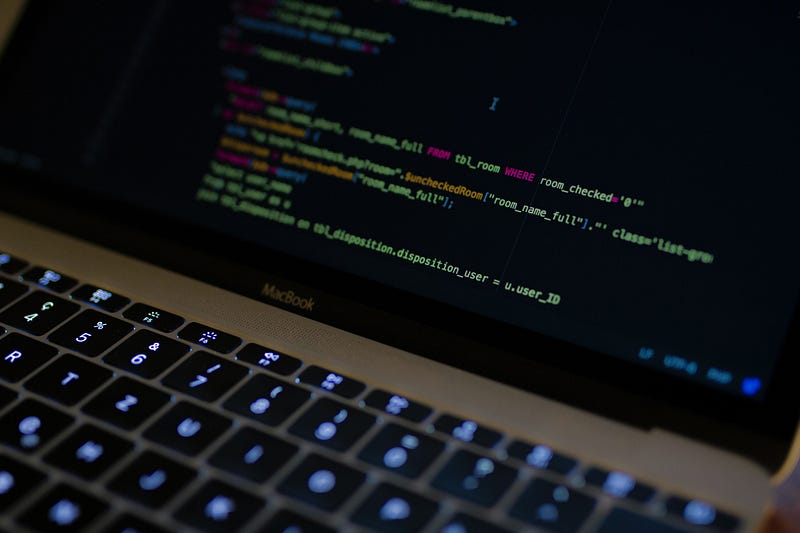
The Most Horrible and Amazing Language: JavaScript!
Oh, pesky JavaScript! How has this language thrived? It has grown-up in the place that we now live … the browser. For most client-side things, it is the only show in town, especially as Flash and Microsoft (I forget it’s name) have both left the stage.
JavaScript is both amazing and absolutely terrible at the same time. And JSON objects? Ahhhh! For this, there’s one little line of code that is essential:
alert(myjson);
So, here’s a little tip I learnt today when debugging with Google Firebase. For this I received the following JSON object when the user uses a Twitter login:
{"user":{"uid":"vxfdsss","displayName":"Fred Smith","photoURL":"https://pbs.twimg.com/profile_images/777/7Nm_normal.jpg","email":null,"emailVerified":false,"phoneNumber":null,"isAnonymous":false,"tenantId":null,"providerData":[{"uid":"226900035","displayName":"Fred Smith","photoURL":"https://pbs.twimg.com/profile_images/777/7Nm_normal.jpg","email":"fred@home","phoneNumber":null,"providerId":"twitter.com"}],...
This contains their email address, photo and unique ID. So let’s unpick it:
{"user":{
"uid":"vxfdsss",
"displayName":"Fred Smith",
"photoURL":"https://pbs.twimg.com/profile_images/777/7Nm_normal.jpg",
"email":null,
"emailVerified":false,
"phoneNumber":null,
"isAnonymous":false,
"tenantId":null,
"providerData":[{"uid":"226900035",
"displayName":"Fred Smith",
"photoURL":"https://pbs.twimg.com/profile_images/777/7Nm_normal.jpg",
"email":"fred@home",
"phoneNumber":null,
"providerId":"twitter.com"}],...
So to read the user’s email address we can use the notation of result.user.email or user[“email”]:
<script>function twitterSignin() {
var provider = new firebase.auth.TwitterAuthProvider();
firebase.auth().signInWithPopup(provider)
.then(function (result) {
var token = result.credential.accessToken;
var user = result.user;
resp = user.email;
Thus we can have result.user.uid, result.user.displayName, and so on. But the email address is “null” in the user part, and where the actual user name is contained in the providerData field. So how do we access that?
Well, to access result.user.providerData gives:
[{"uid":"226900035",
"displayName":"Fred Smith",
"photoURL":"https://pbs.twimg.com/profile_images/777/7Nm_normal.jpg",
"email":"fred@home",
"phoneNumber":null,
"providerId":"twitter.com"}],... }]
But, look, there’s a square backet in front of the JSON object. We can’t just use it as we have done before. What this signifies, is that we have an array object. So we actually need to access it with
result.user.providerData[0]
And now showing this gives:
{"uid":"226900035",
"displayName":"Fred Smith",
"photoURL":"https://pbs.twimg.com/profile_images/777/7Nm_normal.jpg",
"email":"fred@home",
"phoneNumber":null,
"providerId":"twitter.com"}],... }
and after this we can access with:
result.user.providerData[0].email
and which show give us “fred@home”, and not “null” (as in the case of result.user.email). If you want to see this in action, try:
var firebaseConfig = { apiKey: "AIzaSyC5NGQ9fha1V5HEUv4hvV0TwlxNcwd7css", authDomain: "asecuritysite.firebaseapp.com"…asecuritysite.com
For JavaScript, well done for building a new world of software … a faster one … a more interactive one … and removing ourselves from a world of Microsoft operating systems and (often) servers.