Password Entropy
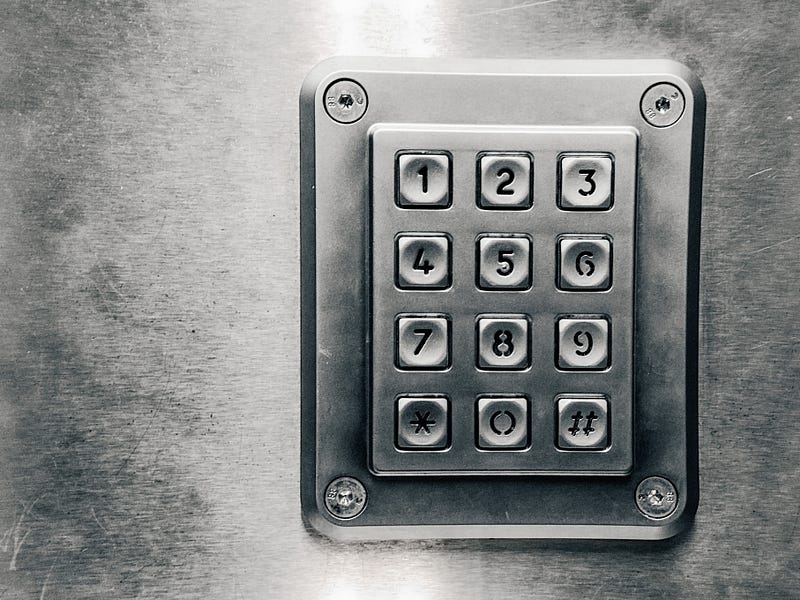
Password Entropy
Password entropy measures the strength of a password and is measured as the number of bits that could represent all of the possibilities. An entropy score of less than 25 identifies a poor password, and between 25 and 50 is a weak password. For 50 to 75 we have a reasonable password, and between 75 and 100 is a very good password. Over 100 is an excellent password.
The strength of the password relates to the number of characters used, and also the number of characters in the password. Character sets include:
'abcdefghijklmnopqrstuvwxyz' (lower case)
'ABCDEFGHIJKLMNOPQRSTUVWXYZ' (upper case)
'0123456789' (numeric)
'!@#$%^&*() (top level characters)
'~`-_=+[]{}\\|;:\'",.<>?/' (additional characters)
If there are N different characters in our character set, and have L characters in the password, the entropy (measured in bits) is:

For “123456”, we have a six-character numeric password and have 10 different characters ( L=10 ) in six positions (N=6). For this we get [here]:

For “qwerty” we have six characters, and 26 possible characters in our character set, so we get an improvement [here]:

For “Qwerty” we have six characters, and 52 possible characters for each one [here]:

For “Qwerty1” we have six characters, and 62 possible characters for each one [here]:

For “Qwerty1!” we have six characters, and 72 possible characters for each one [here]:

For “Qwerty1!~” we have six characters, and 86 possible characters for each one [here]:

In Python, we can use:
import math
L=9
N=96
print (L*math.log(N)/math.log(2))
59.2646625064904
A password that has an entropy value of less than 72 bits can be defined as being crackable. The code in Node.js is:
var entropy = require('string-entropy');
const args = process.argv.slice(1);
mystr = args[2];
console.log("String:\t\t",mystr);
res=entropy(mystr)
console.log("Entropy:\t",res);
if (res<25) console.log("Poor password");
else if (res<50) console.log("Weak password");
else if (res<75) console.log("Reasonable password");
else if (res<100) console.log("Very good password");
else console.log("Excellent password");
Conclusions
And so password strength is related to the number of characters used, and the length of the password. The current level of cracking passwords of GPUs is around 72 bits of entropy, so anything less than that could be cracked in a reasonable time. If you are interested, here’s an estimation of cracking time: