Signcryption: Signing and Encrypting
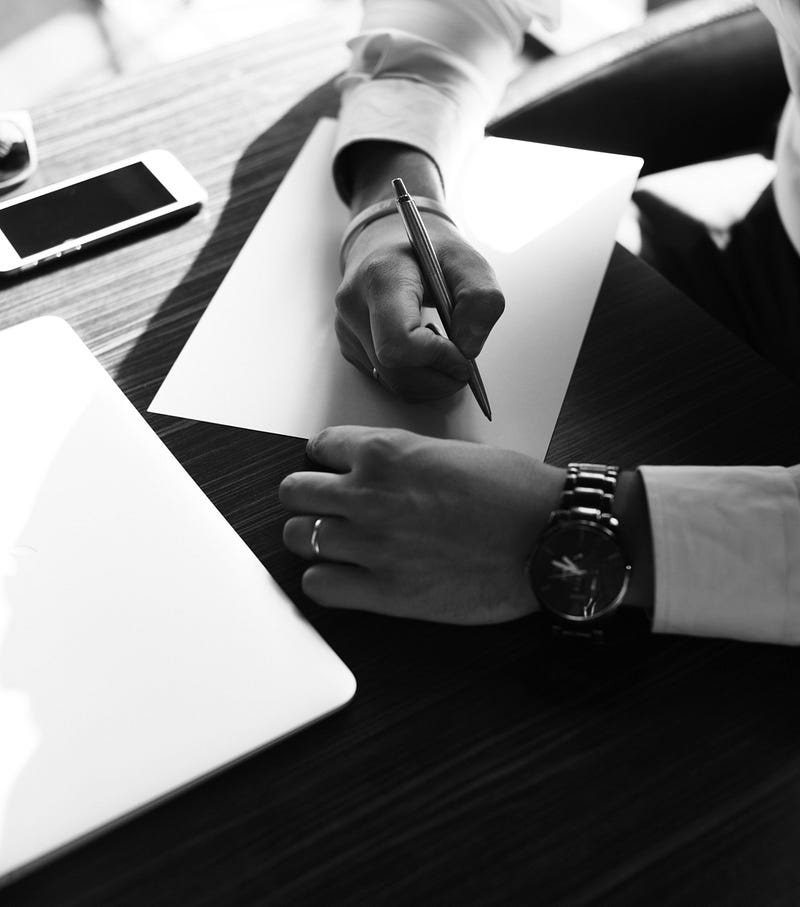
Signcryption: Signing and Encrypting
Normally, when we digitally sign something, we have to create a hash of the data, and then use the public key of the receipitant to encrypt it. So can we sign something at the same time as we encrypt it? Well, we can, and one of the first papers to define this was created by Yuliang Zheng [here]:

One interesting method was created by John Malone-Lee [here][1]:

The method basically uses crypto pairing, and uses crypto pairing mapping of:

And where we use a mapping of one elliptic curve, to another, and then to produce another elliptic curve.
Method
With Signcryption, we can use the Malone-Lee signcryption method [here]. In this case we have a PKG (Private Key Generator), and which generate the private key for Bob and Alice. Bob will have an ID of IDb and Alice will have an ID of IDa.
For the key generation part, the PKG computes the private key of dID and is able to send this to Bob and Alice. Now Alice wants to send a message (M) to Bob, and she computes:

Now Bob decrypts with:

If the Bob’s stored secret is s, his public key is:

Alice gets Bob’s ID (IDb) and then maps onto 𝔾𝟙:

Alice initially creates a random number (x) and takes a base point on 𝔾𝟙 to compute:

We now generate a random number, and within the field of q:

We now calculate:

The ciphertext is then:

When Bob receives this, he takes Alice’s ID and maps her ID onto 𝔾𝟙:

Next he calculates the same value as Alice:

Bob should have the same value of K2 as K1. The message is recovered from:

Coding
The outline coding using the library from the MIRACL library [here] is:
package main
import (
"fmt"
"os"
"github.com/miracl/core/go/core"
"github.com/miracl/core/go/core/BN254"
)
func FP12toByte(F *BN254.FP12) []byte {
const MFS int = int(BN254.MODBYTES)
var t [12 * MFS]byte
F.ToBytes(t[:])
return (t[:])
}
func main() {
rng := core.NewRAND()
var raw [100]byte
for i := 0; i < 100; i++ {
raw[i] = byte(i + 1)
}
rng.Seed(100, raw[:])
AliceID := "Alice"
argCount := len(os.Args[1:])
if argCount > 1 {
AliceID = os.Args[1]
}
q := BN254.NewBIGints(BN254.CURVE_Order)
x := BN254.Randomnum(q, rng)
s := BN254.Randomnum(q, rng)
sh := core.NewHASH256()
for i := 0; i < len(AliceID); i++ {
sh.Process(AliceID[i])
}
QIDb := sh.Hash()
P := BN254.ECP2_generator()
Ppub := BN254.G2mul(P, s)
qIDb := BN254.ECP_mapit(QIDb)
dIDb := BN254.G1mul(qIDb, s)
fmt.Printf("\n==== Trust server:\n")
fmt.Printf("\nSecret: %s\n", s.ToString())
fmt.Printf("\nAlice ID: %s\n", AliceID)
fmt.Printf("Alice Pub (Ppub)= sP:\t%s\n", (Ppub.ToString()))
fmt.Printf("\n=== Bob computes:\n")
U := BN254.G2mul(P, x)
k1 := BN254.Ate(Ppub, qIDb)
k1 = BN254.Fexp(k1)
k1 = BN254.GTpow(k1, x)
fmt.Printf("x: %s\n", x.ToString())
fmt.Printf("U = xP:\t\t%s\n", (U.ToString()))
fmt.Printf("\n\nBob Key (first 20 bytes):\t0x%x\n", FP12toByte(k1)[:20])
fmt.Printf("\n==== Alice computes:\n\n")
k2 := BN254.Ate(U, dIDb)
k2 = BN254.Fexp(k2)
fmt.Printf("Alice Key (first 20 bytes):\t0x%x\n", FP12toByte(k2)[:20])
}
A sample run:
==== Trust server:
Secret: 12789545bf0471f7ba7ac861c7d8faa7b602d14c7fb14090536c712be303603c
Alice ID: Alice
Alice Pub (Ppub)= sP: ([1c1c97cdf2dc0b6e4c055b352582accb105363d3d4913940fbe2663ffdf848f3,0b4d0aa6afcdab183feea20baf783b1eded566b1e3bc1c53e639d5064aca99c6],[1924f2a4966837b91c94dd420b475b29469b01bd675881e3e3373d8fda081f64,13beb94a2b49386c64188c782ea76b4b593ab1f0e4ad496ae65a4f888d5ae817])
=== Bob computes:
x: 04581ca925de0ac1755a9ecbbd2e3458e679f76e4fc95909dc64b49374a7e838
U = xP: ([142b192976ac68eef8f44ed4548359d742e628edad26dee4fa4ae747c24408e3,118796d2d3b0aab3eb3a4d9a821144d606b0861831b393181e05f81bc1550056],[0974d62cf8646c09e15675a4575656783390f2202c71a2e00f22225d2b338dc5,217b26ddfc5d6965e2495babb5a96b92b58eb587783afcbf57c90f3fd977ec92])
Bob Key (first 20 bytes): 0x10724fece94733988c851f2f2f10a3fc6ec2e142
==== Alice computes:
Alice Key (first 20 bytes): 0x10724fece94733988c851f2f2f10a3fc6ec2e142
The coding is here:
https://asecuritysite.com/pairing/mir_signc
Conclusions
In IoT applications, especially for sensor network we need to be efficient in our processor. Signcryption is now being applied into areas of wireless sensor networks:
- Hussain, S., Ullah, S. S., Uddin, M., Iqbal, J., & Chen, C. L. (2022). A comprehensive survey on signcryption security mechanisms in wireless body area networks. Sensors, 22(3), 1072.
- Saravanakumar, P., Sundararajan, T. V. P., Dhanaraj, R. K., Nisar, K., Memon, F. H., Ibrahim, A., & Ag, A. B. (2022). Lamport Certificateless Signcryption Deep Neural Networks for Data Aggregation Security in WSN. Intelligent Automation & Soft Computing, 33(3).
- Gong, B., Wu, Y., Wang, Q., Ren, Y. H., & Guo, C. (2022). A secure and lightweight certificateless hybrid signcryption scheme for Internet of Things. Future Generation Computer Systems, 127, 23–30.
References
[1] Malone-Lee, J. (2002). Identity-based signcryption. Cryptology ePrint Archive.
Asecuritysite is provided free and without adverts. If you want to support its development and get full access to this blog, subscribe here: