What’s So Special About 79be667ef9dcbbac55a06295ce870b07029bfcdb2dce28d959f2815b16f81798?
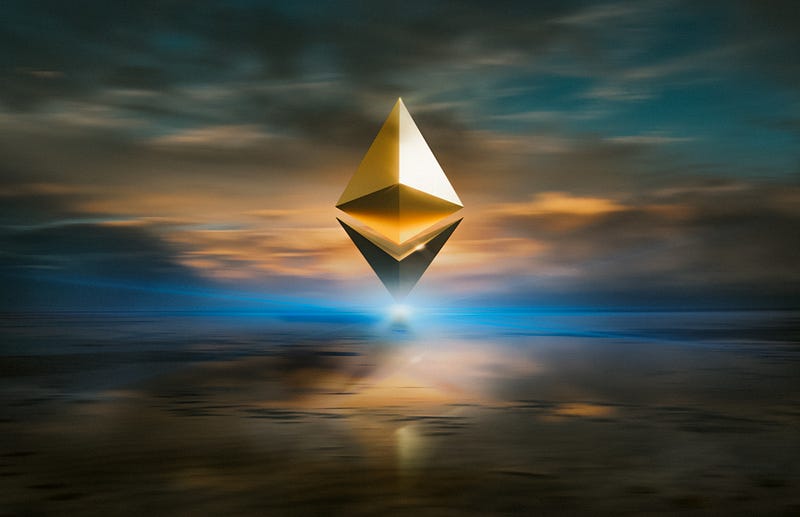
What’s So Special About 79be667ef9dcbbac55a06295ce870b07029bfcdb2dce28d959f2815b16f81798?
One of the most important and interesting areas of cybersecurity is elliptic curve cryptography (ECC). Basically, it protects you like no other method on the Internet. In the creation of the secure tunnel between you and this article, there is likely to be a key negotiation using ECDH (Elliptic Curve Diffie Hellman), and in digitally signing something online, it’s likely that there was an ECDSA (Elliptic Curve Digital Signature Algorithm) signature involved.
At the core of ECC, we have a number of parameters: a, b, p, n and G. a and b define the parameters of the elliptic curve that we use, p is the prime number, n is the order of the curve (the number of points it has), and G is the base point:
In Bitcoins, Satoshi Nakamoto selected the sepc256k1 curve, and where the value of a is your private key (and used to sign for transactions with ECDSA), and aG is your public key. But, what’s G for each curve? Well, we can determine that by just using an a value of 1. This will give us P=1G.
The following is an implementation for a range of curves (Ed25519, BLS 12377, sepc256k1, P256 and Pallas) [here]:
package main
import (
"fmt"
"os"
"strconv"
"github.com/coinbase/kryptology/pkg/core/curves"
)
func main() {
val1 := 1
argCount := len(os.Args[1:])
if argCount > 0 {
val1, _ = strconv.Atoi(os.Args[1])
}
curve := curves.ED25519()
x := curve.Scalar.New(val1)
G := curve.Point.Generator()
xG := curve.Point.Generator().Mul(x)
fmt.Printf("x=%x\n", x.Bytes())
fmt.Printf("\n=== ED25519:\n G=%x\n xG=%x\n xG(x,y)=%x\n", G.ToAffineCompressed(), xG.ToAffineCompressed(), xG.ToAffineUncompressed())
fmt.Printf(" x=%x, y=%x\n \n", xG.ToAffineUncompressed()[0:32], xG.ToAffineUncompressed()[32:])
curve = curves.BLS12377G1()
x = curve.Scalar.New(val1)
G = curve.Point.Generator()
xG = curve.Point.Generator().Mul(x)
fmt.Printf("\n=== BLS12377G1:\n G=%x\n xG=%x\n xG(x,y)=%x\n", G.ToAffineCompressed(), xG.ToAffineCompressed(), xG.ToAffineUncompressed())
curve = curves.K256()
x = curve.Scalar.New(val1)
G = curve.Point.Generator()
xG = curve.Point.Generator().Mul(x)
fmt.Printf(" x=%x, y=%x\n \n", xG.ToAffineUncompressed()[0:32], xG.ToAffineUncompressed()[32:])
fmt.Printf("\n=== Sepc256k1:\n G=%x\n xG=%x\n xG(x,y)=%x\n", G.ToAffineCompressed(), xG.ToAffineCompressed(), xG.ToAffineUncompressed())
fmt.Printf(" x=%x, y=%x\n \n", xG.ToAffineUncompressed()[1:33], xG.ToAffineUncompressed()[33:])
curve = curves.P256()
x = curve.Scalar.New(val1)
G = curve.Point.Generator()
xG = curve.Point.Generator().Mul(x)
fmt.Printf("\n=== P256:\n G=%x\n xG=%x\n xG(x,y)=%x\n", G.ToAffineCompressed(), xG.ToAffineCompressed(), xG.ToAffineUncompressed())
fmt.Printf(" x=%x, y=%x\n \n", xG.ToAffineUncompressed()[1:33], xG.ToAffineUncompressed()[33:])
curve = curves.PALLAS()
x = curve.Scalar.New(val1)
G = curve.Point.Generator()
xG = curve.Point.Generator().Mul(x)
fmt.Printf("\n=== PALLAS:\n G=%x\n xG=%x\n xG(x,y)=%x\n", G.ToAffineCompressed(), xG.ToAffineCompressed(), xG.ToAffineUncompressed())
}
A sample run for a=1 gives [here]:
x=0100000000000000000000000000000000000000000000000000000000000000
=== ED25519:
G=5866666666666666666666666666666666666666666666666666666666666666
xG=5866666666666666666666666666666666666666666666666666666666666666
xG(x,y)=1ad5258f602d56c9b2a7259560c72c695cdcd6fd31e2a4c0fe536ecdd33669215866666666666666666666666666666666666666666666666666666666666666
x=1ad5258f602d56c9b2a7259560c72c695cdcd6fd31e2a4c0fe536ecdd3366921,
y=5866666666666666666666666666666666666666666666666666666666666666
=== BLS12377G1:
G=a08848defe740a67c8fc6225bf87ff5485951e2caa9d41bb188282c8bd37cb5cd5481512ffcd394eeab9b16eb21be9ef
xG=a08848defe740a67c8fc6225bf87ff5485951e2caa9d41bb188282c8bd37cb5cd5481512ffcd394eeab9b16eb21be9ef
xG(x,y)=008848defe740a67c8fc6225bf87ff5485951e2caa9d41bb188282c8bd37cb5cd5481512ffcd394eeab9b16eb21be9ef01914a69c5102eff1f674f5d30afeec4bd7fb348ca3e52d96d182ad44fb82305c2fe3d3634a9591afd82de55559c8ea6
x=0479be667ef9dcbbac55a06295ce870b07029bfcdb2dce28d959f2815b16f817,
y=98483ada7726a3c4655da4fbfc0e1108a8fd17b448a68554199c47d08ffb10d4b8
=== Sepc256k1:
G=0279be667ef9dcbbac55a06295ce870b07029bfcdb2dce28d959f2815b16f81798
xG=0279be667ef9dcbbac55a06295ce870b07029bfcdb2dce28d959f2815b16f81798
xG(x,y)=0479be667ef9dcbbac55a06295ce870b07029bfcdb2dce28d959f2815b16f81798483ada7726a3c4655da4fbfc0e1108a8fd17b448a68554199c47d08ffb10d4b8
x=79be667ef9dcbbac55a06295ce870b07029bfcdb2dce28d959f2815b16f81798,
y=483ada7726a3c4655da4fbfc0e1108a8fd17b448a68554199c47d08ffb10d4b8
=== P256:
G=036b17d1f2e12c4247f8bce6e563a440f277037d812deb33a0f4a13945d898c296
xG=036b17d1f2e12c4247f8bce6e563a440f277037d812deb33a0f4a13945d898c296
xG(x,y)=046b17d1f2e12c4247f8bce6e563a440f277037d812deb33a0f4a13945d898c2964fe342e2fe1a7f9b8ee7eb4a7c0f9e162bce33576b315ececbb6406837bf51f5
x=6b17d1f2e12c4247f8bce6e563a440f277037d812deb33a0f4a13945d898c296,
y=4fe342e2fe1a7f9b8ee7eb4a7c0f9e162bce33576b315ececbb6406837bf51f5
=== PALLAS:
G=0100000000000000000000000000000000000000000000000000000000000080
xG=0100000000000000000000000000000000000000000000000000000000000080
xG(x,y)=0100000000000000000000000000000000000000000000000000000000000000bb2aedca237acf1971473d33d45b658f54ee7863f0a9df537c93120aa3b5741b
With secp256k1 — the Bitcoin and Ethereum curve — we get the following for the base point:
G=0279be667ef9dcbbac55a06295ce870b07029bfcdb2dce28d959f2815b16f81798
xG=0279be667ef9dcbbac55a06295ce870b07029bfcdb2dce28d959f2815b16f81798
xG(x,y)=0479be667ef9dcbbac55a06295ce870b07029bfcdb2dce28d959f2815b16f81798483ada7726a3c4655da4fbfc0e1108a8fd17b448a68554199c47d08ffb10d4b8
In the code, this is generated by:
fmt.Printf("\n=== Sepc256k1:\n G=%x\n xG=%x\n xG(x,y)=%x\n", G.ToAffineCompressed(), xG.ToAffineCompressed(), xG.ToAffineUncompressed())
fmt.Printf(" x=%x, y=%x\n \n", xG.ToAffineUncompressed()[1:33], xG.ToAffineUncompressed()[33:])
For this, the Affine compressed point just gives us the x co-ordinate point, while the Affine uncompressed point gives us the (x,y) co-ordinate point. The ‘04’ part identifies an (x,y) point, and x=79be667ef9dcbbac55a06295ce870b07029bfcdb2dce28d959f2815b16f81798, y=483ada7726a3c4655da4fbfc0e1108a8fd17b448a68554199c47d08ffb10d4b8. We have 64 hex characters for each coordinate value, and thus we have 256-bit x and y values. The full point will thus have 512 bits.
And thus the base point of secp256k1 (the Bitcoin/Ethereum curve) is:
x=79be667ef9dcbbac55a06295ce870b07029bfcdb2dce28d959f2815b16f81798, y=483ada7726a3c4655da4fbfc0e1108a8fd17b448a68554199c47d08ffb10d4b8
You can check here.