How Do You Change Pounds Into Dollars within Encrypted Messages?
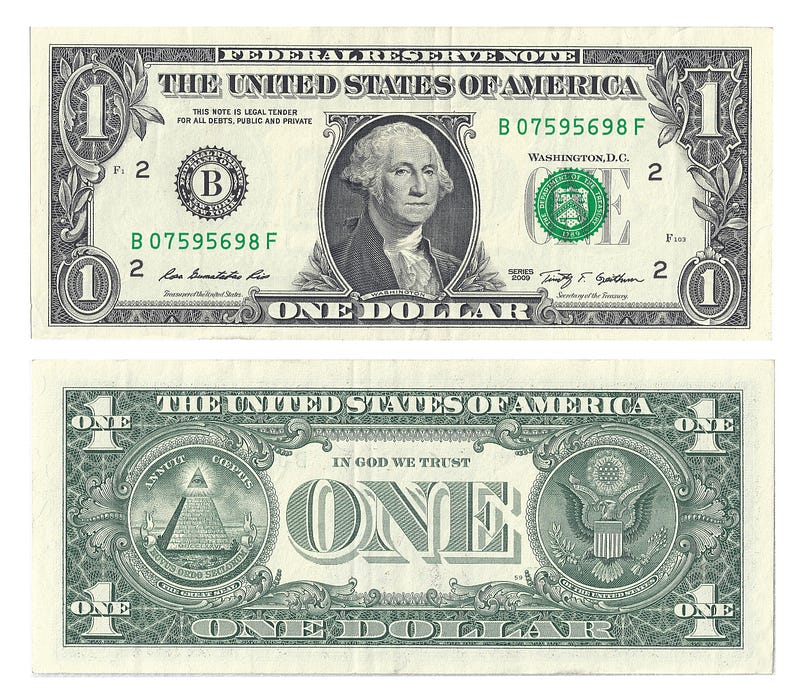
How Do You Change Pounds Into Dollars within Encrypted Messages?
I’ve been demoing bit-flipping in AES [here], and where we need to take a character from the plain text and convert them into another letter by flipping some bits. But, what if we want to change the words? For this, we need to take the plaintext message and X-OR with the words we want in order to get a mask that we can XOR the ciphertext with. So, let’s say we have the message of:
Pay Bob 2 Pounds
and change to:
Pay Bob 2 Dollar
In this, we must convert the cipher for “Pounds” to “Dollar”, and for this we must create a mask which is EX-ORed with the ciphertext. So, let’s try som code:
import sys
import binascii
msg1="Pay Bob 2 Pounds"
msg2="Pay Bob 2 Dollar"
if len(msg1)!=len(msg2):
print("Messages need to be the same")
sys.Exit()
m1=bytearray(msg1.encode())
m2=bytearray(msg2.encode())
i=0
str=""
print(f"{msg1}\t{binascii.hexlify(m1).decode()}")
print(f"{msg2}\t{binascii.hexlify(m2).decode()}")
for ch in m1:
mask=ch^m2[i]
str=str+f"{mask:02x}"
i=i+1
print(f"Mask:\t{str}")
In this case, we get:
Pay Bob 2 Pounds 50617920426f62203220506f756e6473
Pay Bob 2 Dollar 50617920426f62203220446f6c6c6172
Mask: 00000000000000000000140019020501
Notice, that the part of the message we do not want to change has a “0”, and which will mean none of the bits at that position will change. For us, the mask to be applied to the ciphertext would then be: “0x00000000000000000000140019020501”. Once we have the mask, it is a fairly simple task to then apply this to the cipher. An outline of bit-flipping is here: