Removing PKI with Self-certified Public Keys

Removing PKI with Self-certified Public Keys
I know the PKI (Public Key Infrastructure) is the core of security on the Internet, but it is one of the least understood methods in cybersecurity and centralises the power of trust to a few privileged entities. It basically centralises trust.
But, in 1984, Adi Shamir proposed an alternative to PKI (Public Key Infrastructure) named IBE (Identity Based Encryption) [1]:
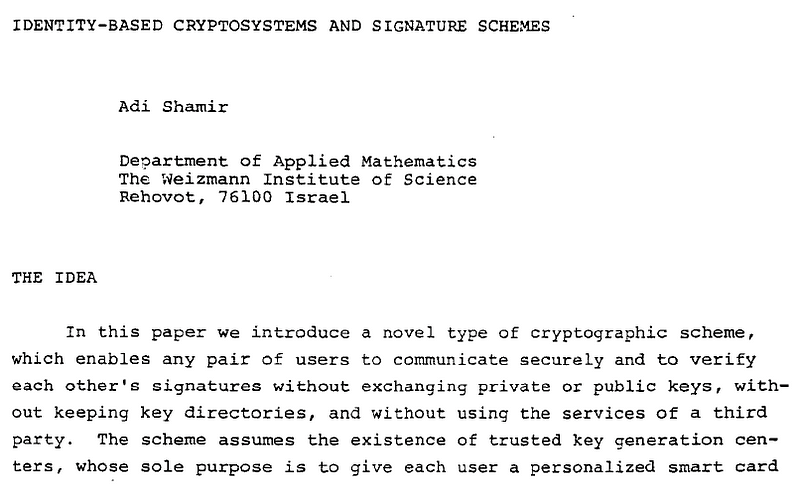
With this, we use the trust centre (T) and which is responsible for generating Bob and Alice’s public and private keys. Anyone who wants to send Alice some encrypted data can generate her public key based on her identity. Girault’s method [2] allows for the trusted server to use an RSA key pair to share a self-certified public key:

The trust centre intially creates two prime numbers (p and q) and calculates the public modulus:

And select an appropriate values for the RSA key pair of (e, d). The trust centre then allocates Alice with a unique distinquishing name (IA) — this could be Alice’s email address. Next Alice chooses a private key of a and then generates a public key for the trust server of:

This is Alice’s key-agreement public key, and where Alice must provide proof to the trust server that she knows the value of a. The trust centre then calculates the reconstruction public data for:

Now Bob takes PA, e, IA and n and can reconstruct Alice’s public key from:

and which should be the same as Alice’s public key of:

This works because:

Alice is the only entity who knows her secret value (a), as the trust centre only knows the public key value for this.
Coding
The coding is [here]:
# https://asecuritysite.com/encryption/girault
from Crypto.Util.number import bytes_to_long, long_to_bytes
from Crypto.Random import get_random_bytes
import Crypto
import libnum
from random import randint
import sys
bits=60
IDA="Alice"
g=2
if (len(sys.argv)>1):
IDA=str(sys.argv[1])
if (len(sys.argv)>2):
bits=int(sys.argv[2])
p = Crypto.Util.number.getPrime(bits, randfunc=get_random_bytes)
q = Crypto.Util.number.getPrime(bits, randfunc=get_random_bytes)
n = p*q
PHI=(p-1)*(q-1)
e=65537
d=libnum.invmod(e,PHI)
a= randint(0, p-1)
keypublic = pow(g,a,n)
m= bytes_to_long(IDA.encode('utf-8'))
Pa = pow((pow(g,a,n) - m),d,n)
print (f"ID={IDA}")
print (f"p={p}")
print (f"q={q}")
print (f"n={n}")
print (f"e={e}")
print (f"d={d}")
print ("\nAlice now generates secret and public key:")
print (f"\nAlice secret (a)={a}")
print (f"\nPa={Pa}")
check = (pow(Pa,e,n)+m) % n
print ("\nBob uses Pa, e, n and IDA to generate Alice's public key")
print (f"\nReconstructed key: {check}")
print (f"Alice's public key: {keypublic}")
if (check==keypublic):
print ("Key are the same!!!")
A sample run of a 60-bit random prime number [here]:
ID=AliceID
p=264503269823565209532794180052271910681
q=174512709827680177874714113719207722167
n=46159182375192430169732491337053209937448557056632565045375090827198887765727
e=65537
d=9230850423566412710446221698634654766535652308694587415312314214613113370913
Alice secret (a)=131775792437443003460453724555643129326
Pa=12249275942899312930638840711641099260772203909414357632461698984354925087146
Bob uses Pa, e, n and IDA to generate Alice's public key
Reconstructed key: 31750704093916555073702705228103148912943576662381570894483735779589546406180
Alice's public key: 31750704093916555073702705228103148912943576662381570894483735779589546406180
Key are the same!!!
Conclusions
Within large-scale IoT network, the concept of a trust centre is now a core part of controlling security. In research, you should never really ignore any valid method because it is not currently used. Thus, to study it is often important in seeing the ways that researchers have solved a problem from the past.
References
[1] Shamir, A. (1984, August). Identity-based cryptosystems and signature schemes. In Workshop on the theory and application of cryptographic techniques (pp. 47–53). Springer, Berlin, Heidelberg [here].
[2] Girault, M. (1991, April). Self-certified public keys. In Workshop on the Theory and Application of of Cryptographic Techniques (pp. 490–497). Springer, Berlin, Heidelberg [here].