When Bob and Alice Have a Secret … They Can Generate Another Secret: Meet J-PAKE

When Bob and Alice Have a Secret … They Can Generate Another Secret: Meet J-PAKE
J-PAKE (Password Authenticated Key Exchange by Juggling) was created by Hao and Ryan [1] and fully defined in RFC 8238 [2][here]:


It is a Password Authentication Key Exchange method, and where Bob and Alice share the same secret password (s). They can then generate a shared secret key. It involves two stages: a one-time key establishment; and a key confirmation stage. Overall, it does not need access to the PKI infrastructure but involves a one-time key establishment and a key confirmation stage.
Round 1
Alice then generates two random values: x1 and x2, and where x1 is a value between 0 and q, and x2 is a value between 0 and q. The values of x_1 and x_2 are kept secret, and where Alice will send the following to Bob:

Alice will also create a Zero Knowledge Proof (ZKP) for x_1, and another for x_2:

This is completed with a zero knowledge proof of x using a Schnorr’s signature, and has two elements g^v and r=v−x.h for x and where v is a random value and h is a non-interactive challenge.
Bob then generates two random values: x_3 and x_4. These are kept secret, and where Bob will send the following to Alice:
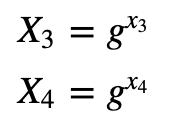
Bob also creates a Zero Knowledge Proof (ZKP) for x3, and another for x4:
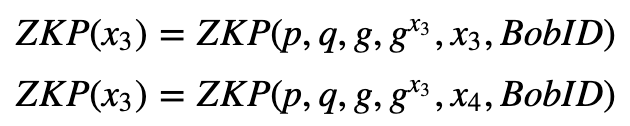
Round 2
Alice then calculates the following and sends to Bob:

Bob then calculates the following and sends to Alice:

Final round
Alice then calculates the shared key as:

Bob then calculates the shared key as:

These keys should be the same. The proof is here:

Coding
First we create a folder named “bc_jpake”, and then go into that folder. We can create a Dotnet console project for .NET 8.0 with:
dotnet new console --framework net8.0
We then add the latest Bouncy Castle library:
dotnet add package BouncyCastle.Cryptography --version 2.2.1
The following is the coding [here]:
namespace JPAke
{
using Org.BouncyCastle.Crypto.Agreement.JPake;
class Program
{
static void Main(string[] args)
{
var bobname="Bob";
var bobpassword="test";
var alicename="Alice";
var alicepassword="test";
if (args.Length >0) bobname=args[0];
if (args.Length >1) bobpassword=args[1];
if (args.Length >2) alicename=args[2];
if (args.Length >3) alicepassword=args[3];
try {
var bob = new JPakeParticipant(bobname, bobpassword.ToArray());
var alice = new JPakeParticipant(alicename, alicepassword.ToArray());
var BobtoSend1 = bob.CreateRound1PayloadToSend();
var AlicetoSend1 = alice.CreateRound1PayloadToSend();
bob.ValidateRound1PayloadReceived(AlicetoSend1);
alice.ValidateRound1PayloadReceived(BobtoSend1);
var BobtoSend2 = bob.CreateRound2PayloadToSend();
var AlicetoSend2 = alice.CreateRound2PayloadToSend();
bob.ValidateRound2PayloadReceived(AlicetoSend2);
alice.ValidateRound2PayloadReceived(BobtoSend2);
var KeyBob= bob.CalculateKeyingMaterial();
var KeyAlice= alice.CalculateKeyingMaterial();
var BobtoSend3 = bob.CreateRound3PayloadToSend(KeyBob);
var AlicetoSend3 = alice.CreateRound3PayloadToSend(KeyAlice);
bob.ValidateRound3PayloadReceived(AlicetoSend3,KeyBob);
alice.ValidateRound3PayloadReceived(BobtoSend3,KeyAlice);
Console.WriteLine("Bob: {0},{1}", bobname,bobpassword);
Console.WriteLine("Alice: {0},{1}", alicename,alicepassword);
Console.WriteLine("\n\n== Shared Secret == ");
Console.WriteLine("Key Bob = {0}",Convert.ToHexString(KeyBob.ToByteArray()));
Console.WriteLine("Key Alice = {0}",Convert.ToHexString(KeyAlice.ToByteArray()));
Console.WriteLine("\n\n === Details ===");
Console.WriteLine("Round 1: Alice X1: {0}",AlicetoSend1.Gx1);
Console.WriteLine("Round 1: Alice X2: {0}",AlicetoSend1.Gx2);
Console.WriteLine("Round 1: Alice Knowledge x1: {0}",AlicetoSend1.KnowledgeProofForX1);
Console.WriteLine("Round 1: Alice Knowledge x2: {0}",AlicetoSend1.KnowledgeProofForX2);
Console.WriteLine("Round 1: Bob X3: {0}",BobtoSend1.Gx1);
Console.WriteLine("Round 1: Bob X4: {0}",BobtoSend1.Gx2);
Console.WriteLine("Round 1: Bob Knowledge x3: {0}",BobtoSend1.KnowledgeProofForX1);
Console.WriteLine("Round 1: Bob Knowledge x3: {0}",BobtoSend1.KnowledgeProofForX2);
Console.WriteLine("Round 2: Alice A: {0}",BobtoSend2.A);
Console.WriteLine("Round 2: Bob B: {0}",AlicetoSend2.A);
} catch (Exception e) {
Console.WriteLine("Error: {0}",e.Message);
if (!bobpassword.Equals(alicepassword)) Console.WriteLine("Alice and Bob's secrets are different");
}
}
}
}
A sample run is [here]:
Bob: Bob,Qwerty123
Alice: Alice,Qwerty123
== Shared Secret ==
Key Bob = 22115BF89A88330D1BAB89FD830F5922DFB9277C54CCA7008866DAC778D37872731CA3A1976377FB69602566D84401C1FF9C0DA741C8C8496701501CC13C5E1EC585EF191221875C7CD197EF7564177AB59E98B9F896E6F706DE851438C2DD016274752957129FDBCBFEEB85FC192600A68E9E67F553A8A799145B966E654F6E0DADB6ED103352643F72C6E175124193763D6665F945AAB0C9F8D4BF2193B0C16EF5F28C1BBC21EEC4FA3462B3B2AAA8F3B4E01BD5047CD72FD7500BBF2033E3F91CFE981ACFB8498D470046E16E6405F5D1AC6F0008F7B660F0177102A7B4322CEFC643A7A3AA838B6937D33505AB2A827BF556BD00334F84355EBC0914E490DFEC7DE69C6BFB5FB1691634C7C227FB49FD1A4965A764E54960B701AD3F18E1D5282C5726273DE8C0F6A6583BACF86752C6C6D2DF36390735751B55BDEE147668BD987CDA5B56FC7D3E59EDDEE5B8D676E61701A619C71F8D09362091179655FACCF186C81ED743C64B31EEAD70EA01BFFDD02B51E4F4BCFC0D158CBDB72F68
Key Alice = 22115BF89A88330D1BAB89FD830F5922DFB9277C54CCA7008866DAC778D37872731CA3A1976377FB69602566D84401C1FF9C0DA741C8C8496701501CC13C5E1EC585EF191221875C7CD197EF7564177AB59E98B9F896E6F706DE851438C2DD016274752957129FDBCBFEEB85FC192600A68E9E67F553A8A799145B966E654F6E0DADB6ED103352643F72C6E175124193763D6665F945AAB0C9F8D4BF2193B0C16EF5F28C1BBC21EEC4FA3462B3B2AAA8F3B4E01BD5047CD72FD7500BBF2033E3F91CFE981ACFB8498D470046E16E6405F5D1AC6F0008F7B660F0177102A7B4322CEFC643A7A3AA838B6937D33505AB2A827BF556BD00334F84355EBC0914E490DFEC7DE69C6BFB5FB1691634C7C227FB49FD1A4965A764E54960B701AD3F18E1D5282C5726273DE8C0F6A6583BACF86752C6C6D2DF36390735751B55BDEE147668BD987CDA5B56FC7D3E59EDDEE5B8D676E61701A619C71F8D09362091179655FACCF186C81ED743C64B31EEAD70EA01BFFDD02B51E4F4BCFC0D158CBDB72F68
=== Details ===
Round 1: Alice X1: 297995405796525790738127322587885304746163450654303320977222603694300622047740930781841779598103496202717976687274513358130865233296556116451333385738347954231372448050058571992461363337852176410390634481539775325098774626520780656161201017272439723441976785419141196302301793301701985440703929813980959141663170781552218285791548352023080435892339189305527558165558999218964659562282907719439229603865737021671691055331438839918247416778491683722576305234081413793514004578008436842440495702592225847570888446160457187709416582595087016939774966457818014396627375170902502118423329665396585846660391684657241347110052615528569150404792162551160126817481565258315006589383571516947546941774202005986770418340122606933590161326959647779345356125467668037456353862166839808920653419625225019174319712029386489795288359216084443400000623679185269937398931588855926044182739206185923785231725608391948499487016726719225917142014
Round 1: Alice X2: 2112113012352189374407950281611172217165960594893662106981084478158480759994056773161922860153312188180335493174036694922964438417944637680528923125075860592963034885812497322550500735935569394795669833937139791332395240175219659891549909189823686208625301926445410143660287301200508952039448717931236590144311078737392804827619970254689457160248428696600693992244047310388609656389517427480774664013935414893557942622876032402469117304104583967025087564502012316397996619436398091878382206162743489041210262930002433633117002526978896610237616505565956900456522370113264931390259798592892714163507610704316679704723594637694597737441507098496180539623143657644410310637632807493637222510185205950718196092261879915749280568582337006637436090946294862206373736569583494215673450520154181070919187181233982641520447410068124888699454690365140845713766482252452101080297845428736680968620843830391122941144469478825218270288640
Round 1: Alice Knowledge x1: 497107990778478053336287055726497955342142734792927355208701392839704498749256579648178139202717103520203303345252930135811570253952850109427901501593735700859552103110805367683063184344353268584186231582627667920973845758025469600445793331343505151390658327009489037579898208553903135926036583166982467770251723452333376735941721401336320063921138506881067073165239793378375647234776098055472055551189165432212941543625333768712167004398393656206906448179516328676854042372081182837867095159556474103543449469687917293064692167692556531748769949735316110594500946617542238600958096515447646623709943674190471206566890953881566975282697068922639263062823643482786858630772254370709003711347048835082965055848467434841125912732108061903472853430558013603169864235478986426090973034916745810350898893918580488543222427588255428199813950306490687673135034929578152608687758953402293759331201499872437130190303249379182918578722
Round 1: Alice Knowledge x2: 1029577835914317701607992666729713498107536301464757088570376293980785904183299349580930998618420430639749509378240805348655242288031254772151164737585670095555094722022038339985380708972545276213692378020387699308852832738937661015110527242895371821946521858356784659251850442017681330102048308447270374088478034789804979023159372113091815608876892657831948144082801379599425801322413299886458979379078016697629892488454915451165037173885048186601712904001477503515239306334091815090858166514186126664000664829604692394987103208155207670487644470103444488482928673366009838031336187063361268121442627746861849169603706309560257727592269624636358566346618235480822277567926646903862187310184538237415385099394146766993654033150432291920753828796039178518642614797189191759445271287683226639682832206541603942629600913452153487284446853010088745850713156808525026935054345348549503309663988199289920349954184102932575487993620
Round 1: Bob X3: 2699531907151805687719204327506947467103270102680829700646407398997525170240186674371076345850259187416964332148255798251975905271213901637104760469185465029746470451544204727142644979571733255510479986596004923762178050149363370942110716941438060234011815529708585816079202995826042430254487925865054523438374751461365924355964621903336253391378432401617216322575545477698370524951642144786661783431402614309271531395145543499033418648595490649386888523762342896700894252682472537551604087433495657509244946325391227217289208061519134820751925991026568152460774691481599652530235524034166257638095759004013942751422831871402600072507788206024189531553687224626191399490099809345784169490163310076462899340468069594966319862951393136821389425297762978316170999690833735065881435441050472145461432418758657779448374703689112354728429967108363538057391007261538886535125146469702602848415876264379824295219655233935668210479862
Round 1: Bob X4: 496962543258721610516184092484659446110284088969996205083960968419228395826671414226249412258905651251324427140308439202112913134266048511744467109960451708950359729480269685331897508227481540017191211349318567115151255550294527653148540623093947971325884787384811015848619054400840497639832073233455268498418455354758225787987925641180841340775722210614385480953890460356179192472061437104780413482193159277936769253592236643850354659941835622384964009039933543765897952850871776257451690607041021498362274646831134171259383395710050869885812198681561688889752714000059620112015110358756711765918012927076642441089751266268776034011045682439422620617029135927040717702144275529446701405603662762604899626644384084341734481987511261562815527949854451897282444646173457525277666511496017450362372082984323650755567386170518881154725212210117569062694191670665104547616794032175273272965673514351076290973171855183046249493632
Round 1: Bob Knowledge x3: 2382690320038848783843192327424225162182479338056609301307465834280125463053795613970326861653937406664938327552765165635969410504236918846008701777577788460525848218696275421137059449555790003478897432946928683880388668370133350886386831485400181676965109525534210967138294572963722490547911774317412838852653450048497230628720974187910844041992423362230631735669561896347893392963682532616367833425646004424198515748703514049781301583409269015847753792488916376297146125433410022670769973527655844500619770017601980161607157443364272442976283999580851262166088915210839813138203108863680590667161628136078836163893807382975252562178646141396232731685589050781434473849862775967354447506480427682788458111999738775680502063041516403146624975061832174367692679249226128936024953623368139280658316526173051101313792646764916078247091923606747368879101601188078769785296767835656354150022688013102085937913076387547213294605483
Round 1: Bob Knowledge x4: 315171161758672906111266478133677044906506178261229825878568263556991982788871562020083280218308746067463133916219716100413716554800642905759426847955786241161677942538561374124769946876732497443627503058062387725287409369630751647911149118880655282139984125989415618818239285757797061982712762908076316551035778783334037812598258298086750455866259220957000096739841358720577237633580294260543071885532493548625763903139375341565521113399599833830641726154745223757220291666109885384842274471607148411180054017557782573449777801472949785460708812843241327627795782901309971342416642618206916414928037753412461476058498985113429922927909102854661350553452190551638898025434506907972678371749818169837096336158823670153471258198593000954734679562733973585385849514183746998132949419491769038088287207409331829268302244763545667124576206202525727285025381157699514896459351459439849309661876047900613517475148772214879516065453
Round 2: Alice A: 2528355648735057773133458420818526716074664581853177242536206120681498510863787451157976978126784121083883818016476098772423982073845060384623154003426404379534731553643168909875623529450797205510852375915013915539663051095609365293641399481434607472674944026565669358732131936342910186262590945225260613539554414159874625097941287202039647001559829929181861324480689650454641970955167968606176703189195659164097672328598570287830482045684877487396249653361242236111876632729241855358109273181956742821833578921223796537102378450392081037608674816245088686928683566102244462076331125466467192972947589724410670212234392377289082113018735482773354578091697826317340466009435135875387937837927434568115252633647006390774738819757731065739614049553311717098240795692413578126536815722921482543149193491541637830836325895249473521197555822120481300813840814874048793433014941175998800976374478890698298556862695564096233534605356
Round 2: Bob B: 1915444897353432048943274092782493721470402383730725751700533541043720672014923823438239631463759226107370651151008814172688896003437028684818037777250019533351272871496861150479405593295101332713258954096175092746078779324881423863273464899054687922615790265975209211092734897058336679453505542742457048465088580982183799904225529249424543256996146624073491488796533171308946740314517086189571163774321171872948261080227589838476810407955547369019088251971289609672905413890662431036029995774328774378894501925022060143304282923174498509391030618004807135874723155860525497655404395857482390370326333265994040980622329694029917945030188629562610584276073516104474315091850852894629862995913530565591870034246314582906476626860949122495138844256049930927840026617763354136875709600633934249840901427399086694281672696453801403729976179093743705327335676467361080052769325779585613974780108013802616572862844507792108874760587
But if Bob and Alice have different secrets [here]:
Error: Partner MacTag validation failed. Therefore, the password, MAC, or digest algorithm of each participant does not match.
Bob and Alice's passwords are different
Conclusions
We could thus use J-PAKE to have two computers which share a secret, and then create a new secret (such as for an encryption key). If you are interested, here are some of the other PAKE methods:
https://asecuritysite.com/pake/
References
[1] Hao, F., & Ryan, P. Y. (2008, April). Password authenticated key exchange by juggling. In International Workshop on Security Protocols (pp. 159–171). Springer, Berlin, Heidelberg [here].
[2] F. Hao, J-PAKE: Password-Authenticated Key Exchange by Juggling. RFC 8236 [here].