RSA Still Rules For Trust on the Internet
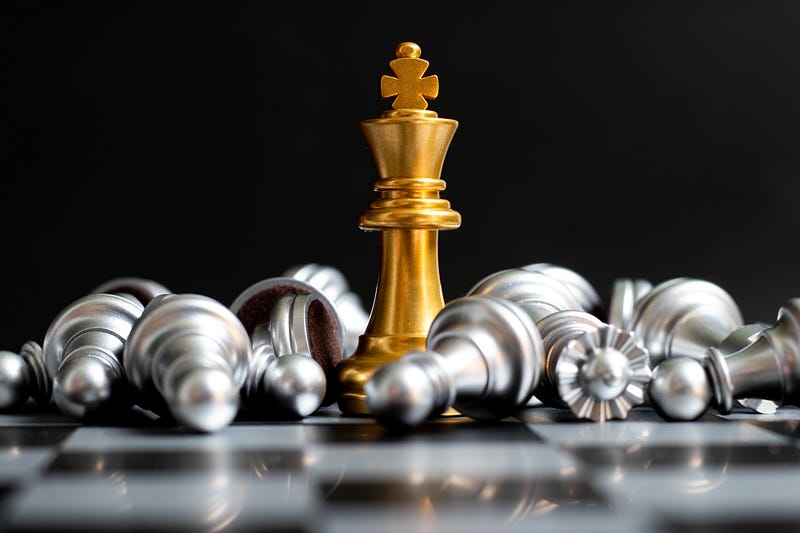
RSA Still Rules For Trust on the Internet
RSA signatures hold up the trust of the Internet, and without it, we could not trust many of the sites we visit. I did a quick survey of the public keys for some sample Web sites, and I got:
- BBC.com: Elliptic Curve 256
- NatWest.com: RSA
- Microsoft.com: RSA 2K
- Cisco.com: RSA 2K
- IBM.com: RSA 2K
- Facebook.com: Elliptic Curve 256
- Apple.com: RSA 2K
- NetFlix.com: Elliptic Curve 256
- Napier.ac.uk: RSA 2K
- Mit.edu: RSA 2K
In this small sample, we can see we have 70% with RSA keys (and with an RSA signature) and 30% with Elliptic Curve keys (and with an ECDSA signature).
With RSA, we have the magic of public key encryption, and where Bob can generate a key pair: a public key and a private key, and then send Alice his public key. If she wants to encrypt something for him, she encrypts it with his public key, and then the only key which can decrypt it is his private key. A truly wonderful concept and it was Rivest, Shamir and Adleman who made it come alive with the RSA method. In RSA, we start by generating two prime numbers (p,q) and then calculate the modulus (N) of N=pq. It is this modulus (N) that provides the core of the security of RSA, and it must be difficult to determine the prime numbers for a given modulus value. Our prime numbers must thus be of a size that makes it difficult to factorize, and they must be randomly generated.
In this case, we will use .NET and Cryptography Next Generation (CNG), and generate different sizes of modulus values, and generate a signature. With RSA, we have two prime numbers (p and q), and compute the modulus (N):
N=pq
The full process is then:

We first convert our message into a byte array, and then create Bob’s key pair [here]:
var msg=Encoding.ASCII.GetBytes(message);
// create Bob's key pair
var bob=new RSACng(size);
We can then hash the message, and create the signature with Bob’s private key [here]:
var h1=SHA256.Create();
var res= h1.ComputeHash(msg);
var sig = bob.SignHash(res,HashAlgorithmName.SHA256,padding);
Finally Alice can import Bob’s public key and then verify the signature [here]:
var alice=new RSACng(size);
alice.ImportFromPem(bob.ExportRSAPublicKeyPem());
var rtn = alice.VerifyHash(res,sig,HashAlgorithmName.SHA256,padding);
The full code [here]:
namespace CngRSA
{
using System.Security.Cryptography;
using System.Text;
class Program
{
static void Main(string[] args)
{
var message="Hello";
var size=512;
var pad="PKCS1";
try {
var padding = RSASignaturePadding.Pkcs1;
if (args.Length >0) message=args[0];
if (args.Length >1) size=Convert.ToInt32(args[1]);
if (args.Length >2) pad=args[2];
if (pad=="Pkcs1") padding = RSASignaturePadding.Pkcs1;
else if (pad=="Pss") padding = RSASignaturePadding.Pss;
var msg=Encoding.ASCII.GetBytes(message);
// create Bob's key pair
var bob=new RSACng(size);
var h1=SHA256.Create();
var res= h1.ComputeHash(msg);
var sig = bob.SignHash(res,HashAlgorithmName.SHA256,padding);
var alice=new RSACng(size);
alice.ImportFromPem(bob.ExportRSAPublicKeyPem());
var rtn = alice.VerifyHash(res,sig,HashAlgorithmName.SHA256,padding);
Console.WriteLine("Size:\t\t\t{0}",size);
Console.WriteLine("Message:\t\t{0}",message);
Console.WriteLine("Padding:\t\t\t{0}",pad);
Console.WriteLine("Hash:\t\t{0}",Convert.ToHexString(res));
Console.WriteLine("Signature:\t\t{0}",Convert.ToHexString(sig));
Console.WriteLine("Verified:\t\t{0}",rtn);
var b = bob.ExportParameters(true);
Console.WriteLine("\nBob Private Key D:\t\t{0}",Convert.ToHexString(b.D));
Console.WriteLine("\nBob Private Key P:\t\t{0}",Convert.ToHexString(b.P));
Console.WriteLine("\nBob Private Key Q:\t\t{0}",Convert.ToHexString(b.Q));
Console.WriteLine("Bob Private Key (DER):\t\t{0}",Convert.ToHexString(bob.ExportPkcs8PrivateKey()));
Console.WriteLine("Bob Private Key (PEM):\n{0}",bob.ExportRSAPrivateKeyPem());
Console.WriteLine("\nBob Public Key e:\t\t{0}",Convert.ToHexString(b.Exponent));
Console.WriteLine("\nBob Public Key N:\t\t{0}",Convert.ToHexString(b.Modulus));
Console.WriteLine("Bob Public Key (DER):\t\t{0}",Convert.ToHexString(bob.ExportSubjectPublicKeyInfo()));
Console.WriteLine("Bob Public Key (PEM):\n{0}",bob.ExportSubjectPublicKeyInfoPem());
} catch (Exception e) {
Console.WriteLine("Error: {0}",e.Message);
Console.WriteLine("PLease use a 1,024 bit key or above for OaepSHA256 and other padding methods.");
}
}
}
}
A sample run with a signature is [here]:
Size: 512
Message: Hello123
Padding: Pkcs1
Hash: 134563D4E440F0E418B0F382F23A2CF301AF6D7F648CCFAE9895018345D779A3
Signature: 91805B04FB5E141B45F2BCDF789BEEEDEFED14FA28CA9BF39BE234ECD6E0D0C2878CA34FC5F124EA652152254FFF04E1F79F0C0E57566382FE1C58F4978DFD2F
Verified: True
Bob Private Key D: 1CB36948B384242312B87A5ABDDF15E75B0C3FEA168981EC44766F4D3B4BF28D35A97033E2E1378073F14E6624CD8EBB23026CFC1E597B75E4DEB1BFF9AAB749
Bob Private Key P: F0ABB4A0268824F48B24464544D5FC65C1604361614CA494EE78C7991C7D5F9B
Bob Private Key Q: CC33BCE3580AAC6BD6EB9A0DB932E61AEE5AA0CC9DD5C46DB986DB2CE0FDEB1F
Bob Private Key (DER): 30820155020100300D06092A864886F70D01010105000482013F3082013B020100024100BFF977B86E47FFC53C2269892D3FB1937013EF3C1B0ECCE35FC02336270371E6E24E033C9CA636F3E2D03CE81BDD655E6667706543FF2F5B2F909BE4A620DCC5020301000102401CB36948B384242312B87A5ABDDF15E75B0C3FEA168981EC44766F4D3B4BF28D35A97033E2E1378073F14E6624CD8EBB23026CFC1E597B75E4DEB1BFF9AAB749022100F0ABB4A0268824F48B24464544D5FC65C1604361614CA494EE78C7991C7D5F9B022100CC33BCE3580AAC6BD6EB9A0DB932E61AEE5AA0CC9DD5C46DB986DB2CE0FDEB1F022100C910AA76A1BA5C2571997C9BA246A3F6DEF76D538DD65A299291952F757DC8C1022100B30AB2154BBEA00B35B801AD02B2E41ABCDE6D9ABAD111977B52089E42EF9FD502204C563E6E37937A4D1C6552E80403C72DC658F0C268A4ED349915DFDCA6D02515
Bob Private Key (PEM):
-----BEGIN RSA PRIVATE KEY-----
MIIBOwIBAAJBAL/5d7huR//FPCJpiS0/sZNwE+88Gw7M41/AIzYnA3Hm4k4DPJym
NvPi0DzoG91lXmZncGVD/y9bL5Cb5KYg3MUCAwEAAQJAHLNpSLOEJCMSuHpavd8V
51sMP+oWiYHsRHZvTTtL8o01qXAz4uE3gHPxTmYkzY67IwJs/B5Ze3Xk3rG/+aq3
SQIhAPCrtKAmiCT0iyRGRUTV/GXBYENhYUyklO54x5kcfV+bAiEAzDO841gKrGvW
65oNuTLmGu5aoMyd1cRtuYbbLOD96x8CIQDJEKp2obpcJXGZfJuiRqP23vdtU43W
WimSkZUvdX3IwQIhALMKshVLvqALNbgBrQKy5Bq83m2autERl3tSCJ5C75/VAiBM
Vj5uN5N6TRxlUugEA8ctxljwwmik7TSZFd/cptAlFQ==
-----END RSA PRIVATE KEY-----
Bob Public Key e: 010001
Bob Public Key N: BFF977B86E47FFC53C2269892D3FB1937013EF3C1B0ECCE35FC02336270371E6E24E033C9CA636F3E2D03CE81BDD655E6667706543FF2F5B2F909BE4A620DCC5
Bob Public Key (DER): 305C300D06092A864886F70D0101010500034B003048024100BFF977B86E47FFC53C2269892D3FB1937013EF3C1B0ECCE35FC02336270371E6E24E033C9CA636F3E2D03CE81BDD655E6667706543FF2F5B2F909BE4A620DCC50203010001
Bob Public Key (PEM):
-----BEGIN PUBLIC KEY-----
MFwwDQYJKoZIhvcNAQEBBQADSwAwSAJBAL/5d7huR//FPCJpiS0/sZNwE+88Gw7M
41/AIzYnA3Hm4k4DPJymNvPi0DzoG91lXmZncGVD/y9bL5Cb5KYg3MUCAwEAAQ==
-----END PUBLIC KEY-----
Conclusions
While elliptic curve methods are making inroads in defining the public key for Web sites, it is RSA that is still them most popular method. Unfortunately, we will have to migrate towards Dilitiu, FALCON or SPHINCS+ over the next few years.
.